TypeScript (TS) is an object-oriented, open-source programming language and an extension of Javascript (JS). It is the superset of JavaScript. Any code written in TypeScript is valid in JavaSript, although the opposite might not be true in some cases. Microsoft developed it in 2012. It follows static typing and is multiparadigm, which means it is object-oriented.
Checking type in TypeScript
The best way to check the type in a TS program is to use the ‘typeof’ operator. ‘typeof’ is an operator that differentiates between types – boolean, string, number, null, etc. In other words, it returns a string that indicates the type of the value and can be used as a type guard in the TS programming language.
JavaScript already uses the typeof operator. Here is an example of how it works in JavaScript:
console.log( typeof "New York City");
This will print the string keyword as the output.
However, in TypeScript, you can add an typeof
operator in a type context to refer to the type of a variable or property. Here is a sample code snippet:
let t = 'Tomato';
let s: typeof t;
Here, we are assigning ‘s’ to be a string.
Also, we can combine this with other type operators and use typeof
it to express several patterns conveniently. For example, let’s start with the predefined type ReturnType<T>
. It takes a function type and gives you its return type:
type Predicate = (x: unknown) => boolean;
type S = ReturnType<Predicate>;
Here, it will print ‘S’ as a boolean.
But, when ReturnType is used on a function name, it will create an error. This will happen because types are different from values, and they are not the same. For example:
function r() {
return { x: 4, y: 20 };
}
type R = ReturnType<r>;
The error message you will get is:
'r' refers to a value, but is being used as a type here. Did you mean 'typeof r'?
This can be written like this instead:
function r() {
return { x: 4, y: 20 };
}
type R = ReturnType<typeof r>;
Thus, we will find out that the function r has x & y, and their type is ‘number’.
There is a catch to using typeof – you can only use it with identifiers, and this is done to avoid any confusion.
We can also use ‘typeof’ with if statements:
const myVar: string | number = 'new york city';
console.log(typeof myVar); // "string"
if (typeof myVar === 'string') {
console.log(myVar.toUpperCase()); // "NEW YORK CITY"
}
It is called Runtime Type Checking. TypeScript only performs static type checking at compile time. Extra runtime checking is done because JavaScript might not understand the types written in TypeScript. Thus, runtime type checking happens.
Conclusion
By using typeof, we can find the type in our TypeScript code. typeof can be used in if statements too. It is essential to know that values and types are different from each other. Use typeof to distinguish between the two while using ReturnType <T>. We can also find the type during runtime, called runtime type checking.
Happy Coding!
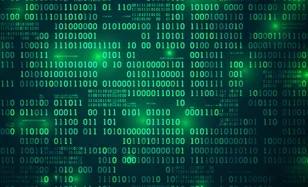